Clustering Methods in Bioinformatics
Spectral Clustering and its applications
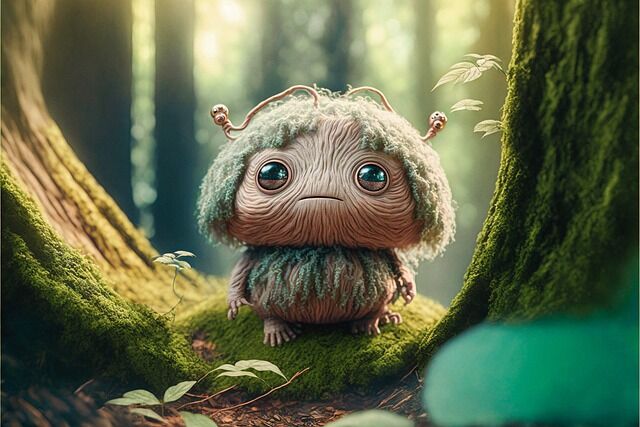
Learning objectives:
Introduction
Overview of Spectral Clustering
Spectral clustering is an unsupervised machine-learning algorithm used for data clustering. It is based on the concept of spectral graph theory and uses the eigenvalues and eigenvectors of a matrix derived from the data points to analyze their similarity.
Spectral clustering aims to partition the data points into clusters based on their similarity. It does this by mapping each data point to a low-dimensional feature space and applying a clustering algorithm to the data points in the feature space.
Spectral clustering is a robust machine-learning algorithm that groups data into clusters or meaningful subgroups based on the concept of graph theory. It has become increasingly popular due to its ability to deal with non-linear data. Spectral clustering is an unsupervised learning method that analyzes the similarity of data points and groups them based on their similarities. This tutorial will cover the basics of spectral clustering, how it works, and how to implement it.
How Does Spectral Clustering Work?
To understand how spectral clustering works, we must first understand the basics of graph theory. A graph is a set of vertices (nodes) connected by edges (links), and the edges represent the relationship between the nodes.
In spectral clustering, each node represents a data point, and the edges represent the similarity between data points. The algorithm then uses the eigenvectors of the graph Laplacian matrix to find clusters in the data. The eigenvectors are vectors that span the graph's space and capture the graph's structural information.
The algorithm begins by constructing a similarity matrix, which is a matrix of the similarities between all pairs of data points. We can accomplish this using various similarity metrics, such as Euclidean distance, Manhattan distance, or cosine similarity.
Once the similarity matrix is calculated, the next step is to calculate the eigenvalues and eigenvectors of the matrix. The eigenvalues and eigenvectors transform the data points into a lower-dimensional feature space. The feature space is then used to identify clusters of data points that are similar.
Finally, a clustering algorithm such as k-means or hierarchical clustering is applied to the feature space to partition the data points into distinct clusters.
An example algorithm for spectral clustering in Python
#import necessary libraries
import numpy as np
from sklearn.cluster import SpectralClustering
#define parameters
n_clusters = 3 #number of clusters
gamma = 1.0 #regularization parameter
#instantiate a spectral clustering model
model = SpectralClustering(n_clusters=n_clusters, gamma=gamma)
#fit the model to the data
model.fit(X)
#predict the cluster labels
labels = model.labels_
#compute the center of each cluster
centroids = np.zeros((n_clusters, X.shape[1]))
for i in range(n_clusters):
points_in_cluster = X[labels == i]
centroids[i] = np.mean(points_in_cluster, axis=0)
#output the cluster labels and centroids
print("Cluster Labels: ", labels)
print("Cluster Centroids: ", centroids)
#Example data set:
#Data point 1: [2.7, 6.1, 3.7]
#Data point 2: [3.9, 5.3, 4.2]
#Data point 3: [4.5, 4.7, 6.2]
#Data point 4: [1.2, 7.4, 5.5]
#Data point 5: [5.5, 3.5, 4.9]
#Data point 6: [6.1, 2.8, 6.3]
Advantages of Spectral Clustering
Spectral clustering has several advantages over other clustering algorithms. For example, it is more efficient than traditional clustering algorithms since it only requires a single pass of the data points to calculate the similarity matrix.
The efficiency makes it ideal for large datasets where the computational time for traditional clustering algorithms can be prohibitively long.
In addition, spectral clustering is not limited to Euclidean distances between data points. It can use any distance metric appropriate for the dataset, making it suitable for datasets with complex relationships between data points.
Furthermore, spectral clustering is robust to outliers and noise in the dataset, resulting in more accurate clusters.
Disadvantages of Spectral Clustering
There are some drawbacks to spectral clustering as well. For example, the number of clusters must be known in advance, and we cannot use the algorithm to compute the optimal number of clusters.
In addition, the algorithm is sensitive to the choice of the similarity matrix and the parameters used to calculate it.
Finally, spectral clustering is computationally intensive and can be slow for large datasets.
Conclusion
Spectral clustering is a robust unsupervised clustering algorithm to partition data points into clusters. It transforms the data points into a lower-dimensional feature space and then applies a clustering algorithm to the feature space. Spectral clustering has several advantages, such as being efficient and robust to noise.
However, it also has some drawbacks, such as requiring the number of clusters to be known in advance and being computationally intensive.